Overview
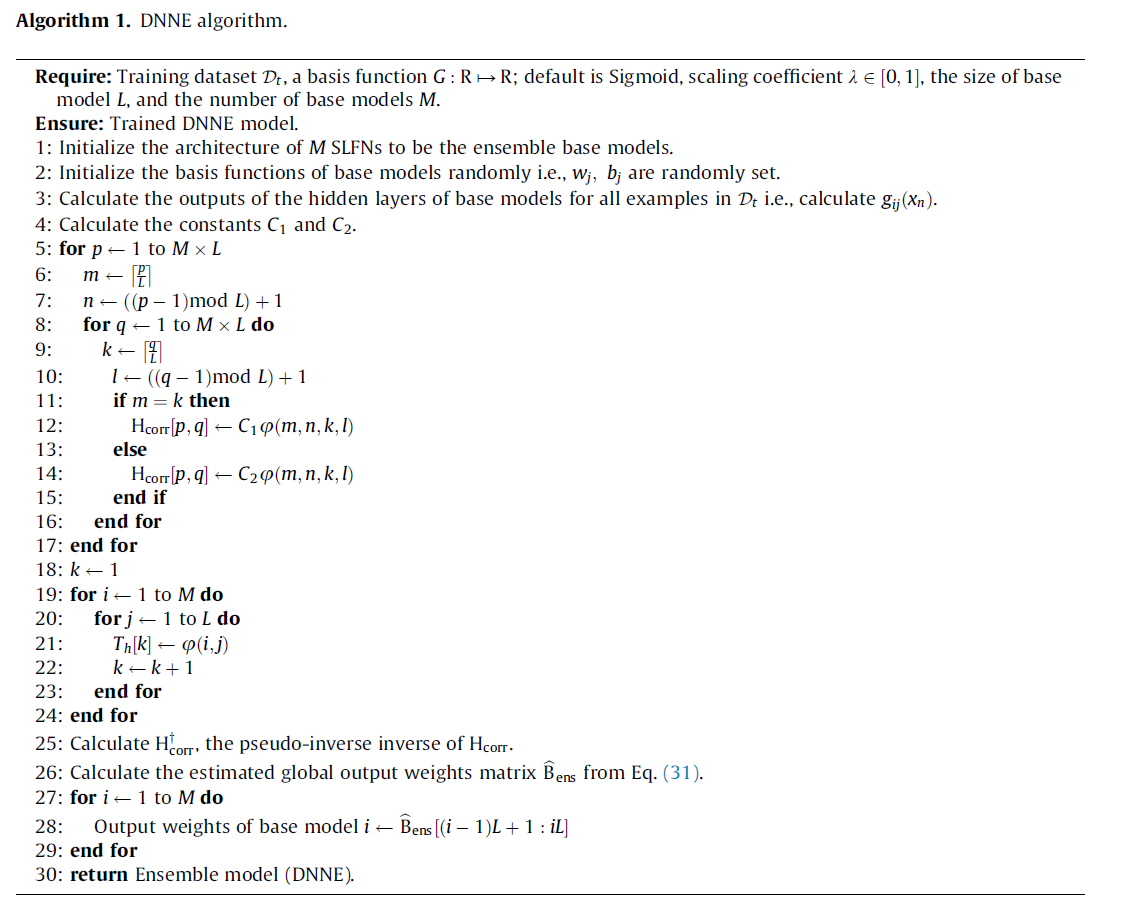
clear all;
data = csvread('data/computer_activity.data');
X = data(:, 2:end);
T = data(:, 1);
dnne = newdnne(5, 70, X, T, 0.5);
[dnne, rmse] = traindnne(dnne, X, T);
netOut = simdnne(dnne, X);
rmse1 = sqrt(sum((T - netOut).^2) / size(T,1));
clear all;
data = csvread('data/calhousing.data');
X = data(:, 2:end);
T = data(:, 1);
dnne = newdnne(5, 50, X, T, 0.55);
[dnne, rmse] = traindnne(dnne, X, T);
netOut = simdnne(dnne, X);
rmse1 = sqrt(sum((T - netOut).^2) / size(T,1));
clear all;
data = csvread('data/credit_german.data');
X = data(:, 2:end);
TOrig = data(:, 1);
noClasses = max(TOrig);
T = ones(size(TOrig,1), noClasses) * -1;
for i=1:noClasses
T(TOrig == i, i) = 1;
end
clear noClasses data i;
dnne = newdnne(5, 100, X, T, 0.55);
[dnne, rmse] = traindnne(dnne, X, T);
predLabels = simdnne(dnne, X, 'class');
acc = sum(TOrig == predLabels) / size(TOrig,1) * 100;
clear all;
data = csvread('data/led_7.data');
X = data(:, 2:end);
TOrig = data(:, 1);
if min(TOrig) == 1
noClasses = max(TOrig);
T = ones(size(TOrig,1), noClasses) * -1;
for i=1:noClasses
T(TOrig == i, i) = 1;
end
elseif min(TOrig) == 0
noClasses = max(TOrig) + 1;
T = ones(size(TOrig,1), noClasses) * -1;
for i=1:noClasses
T(TOrig == i - 1, i) = 1;
end
end
clear noClasses data i;
dnne = newdnne(5, 50, X, T, 0.55);
[dnne, rmse] = traindnne(dnne, X, T);
predLabels = simdnne(dnne, X, 'class');
if min(TOrig) == 1
predLabels = simdnne(dnne, X, 'class');
elseif min(TOrig) == 0
predLabels = simdnne(dnne, X, 'class') - 1;
end
acc = sum(TOrig == predLabels) / size(TOrig,1) * 100;
clear all;
s = RandStream('mt19937ar','Seed',1986);
for i=1:10
% California Housing Dataset (REGRESSION)
data = csvread('data/calhousing.data');
indexes = randperm(s, size(data,1));
t = ceil(0.60 * size(data,1));
trainData = data(indexes(1:t), :);
testData = data(indexes(t+1:end), :);
[housing_dnneModel{i}, housing_trnAcc(i), housing_tstAcc(i), housing_rmse(i)] = dnne(5, 50, 0.55, trainData, testData, 'reg', s);
% German Credit Card Dataset (CLASSIFICATION)
data = csvread('data/credit_german.data');
indexes = randperm(s, size(data,1));
t = ceil(0.60 * size(data,1));
trainData = data(indexes(1:t), :);
testData = data(indexes(t+1:end), :);
[credit_dnneModel{i}, credit_trnAcc(i), credit_tstAcc(i), credit_rmse(i)] = dnne(5, 100, 0.55, trainData, testData, 'class', s);
end
clear data trainData testData s indexes t i
This software package has been developed based on the paper
M. Alhamdoosh and D. H. Wang, Fast
decorrelated neural network ensembles with random weights, Information
Sciences, Vol. 264, pp. 104-117, 2014.
For technical support and/or help, please contact m.hamdoosh
[at] gmail [dot] com